If you want to describe Kafka Consumer group in Java, you may want to use this method:
// returns list of consumers in given consumer group mapped on distributed partitions
public String consumerGroupDescription(KafkaAdmin kafkaAdmin, String consumerGroup) {
DescribeConsumerGroupsResult result = kafkaAdmin.describeConsumerGroups(Collections.singleton(consumerGroup));
ConsumerGroupDescription groupDescription;
try {
groupDescription = result.describedGroups().get(consumerGroup).get();
} catch (InterruptedException | ExecutionException e) {
throw new RuntimeException(e);
}
Collection<MemberDescription> membersDescription = groupDescription.members();
Map<String, Integer> consumerPartitions = new HashMap<>();
for (MemberDescription md : membersDescription) {
consumerPartitions.put(md.consumerId(), md.assignment().topicPartitions().size());
}
StringBuilder sb = new StringBuilder();
for (Map.Entry<String, Integer> entry : consumerPartitions.entrySet()) {
sb.append("\n[").append(entry.getKey()).append("]:[").append(entry.getValue()).append("]");
}
if (sb.toString().equals("")) {
sb.append("<no active members found>");
}
return String.format("----- Group %s description: %s", consumerGroup, sb.toString());
}
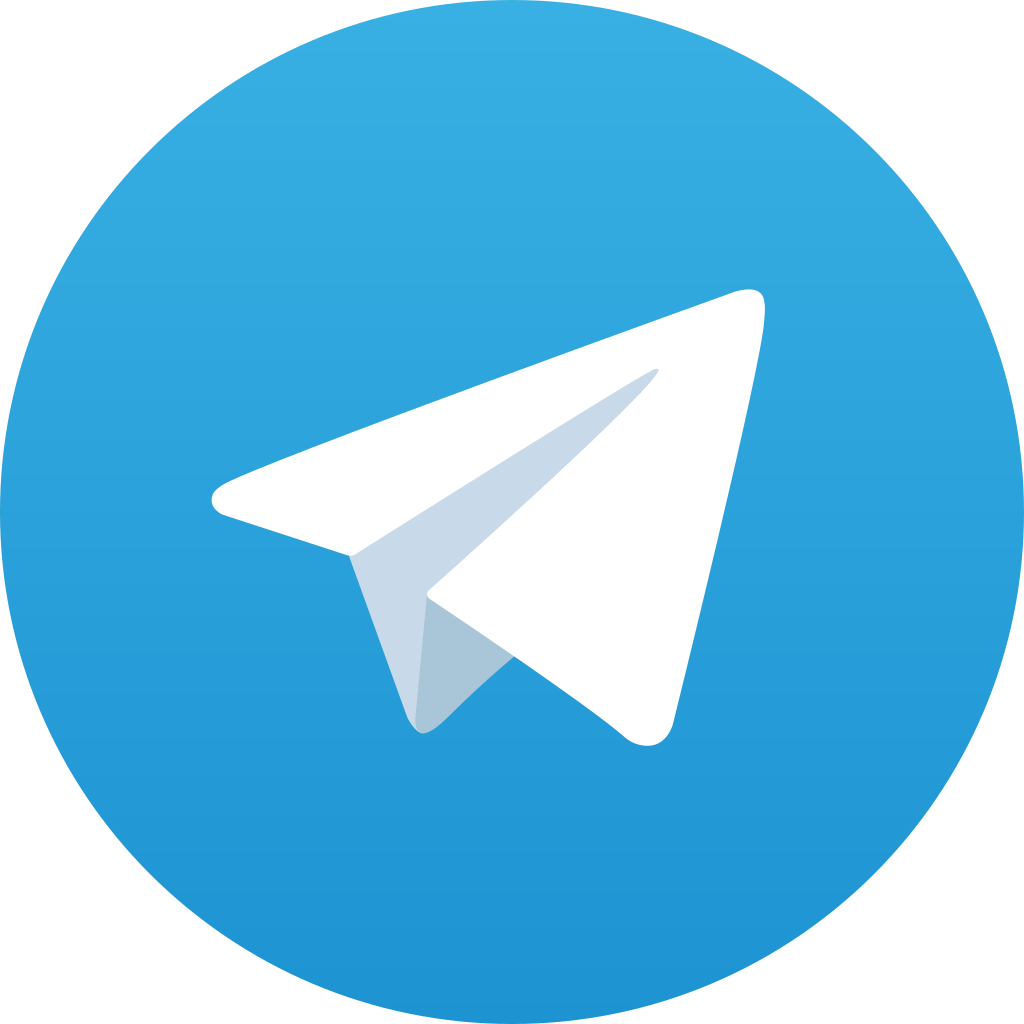
If you still have any questions, feel free to ask me in the comments under this article or write me at promark33@gmail.com.
If I saved your day, you can support me 🤝