Let’s imagine that we have a task:
public class Task implements Runnable {
@Override
public void run() {
..
}
}
And the code for its execution:
ExecutorService executor = Executors.newSingleThreadExecutr();
executor.execute(new Task());
And we need to get the return value after completing the task. For this, Runnable must be replaced with Callable:
public class Task implements Callable<Object> {
@Override
public Object call() {
...
return "return_data!";
}
}
And when sending a task to an ExecutorService, you need to use submit method instead of execute, and capture a Future<Object> object:
Future<Object> future = executor.submit(new Task());
...
System.out.println("return value = " + future.get().toString());
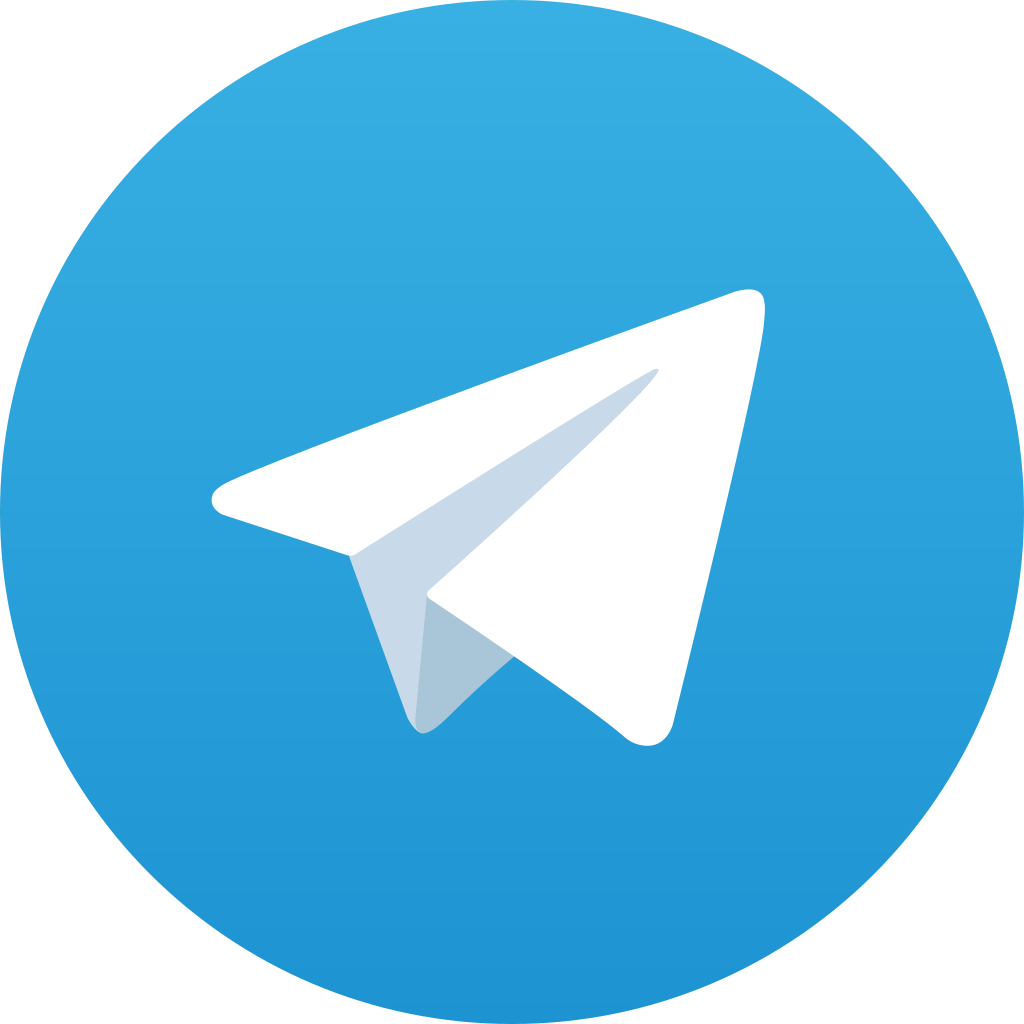
If you still have any questions, feel free to ask me in the comments under this article or write me at promark33@gmail.com.
If I saved your day, you can support me 🤝