title: "my awesome conf"
childConfiguration:
message: "hello world"
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-yaml</artifactId>
<version>${jackson.version}</version>
</dependency>
public class RootConfiguration {
private ChildConfiguration childConfiguration;
private String title;
public ChildConfiguration getChildConfiguration() {
return childConfiguration;
}
public void setChildConfiguration(ChildConfiguration childConfiguration) {
this.childConfiguration = childConfiguration;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public String toString() {
return "RootConfiguration{" +
"childConfiguration=" + childConfiguration +
'}';
}
}
public class ChildConfiguration {
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
@Override
public String toString() {
return "ChildConfiguration{" +
"message=" + message +
'}';
}
}
public RootConfiguration getConfiguration() throws IOException {
ObjectMapper yamlMapper = new ObjectMapper(new YAMLFactory());
File configFile = new File("configuration.yml");
Preconditions.checkArgument(configFile.exists(), "configuration file doesn't exist");
return yamlMapper.readValue(configFile, RootConfiguration.class);
}
If you got MismatchedInputException:
com.fasterxml.jackson.databind.exc.MismatchedInputException
Then visit this link: https://mchesnavsky.tech/jackson-mismatched-input-exception/.
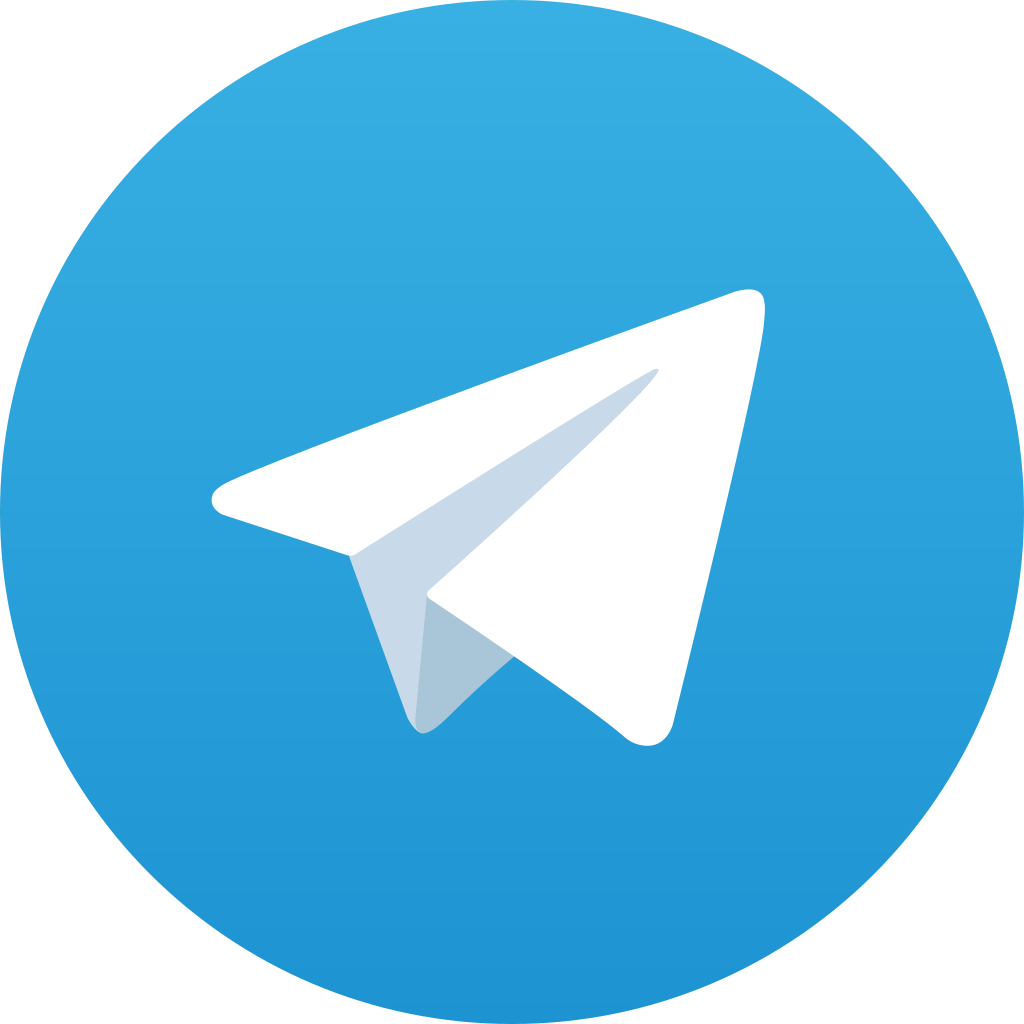
If you still have any questions, feel free to ask me in the comments under this article or write me at promark33@gmail.com.
If I saved your day, you can support me 🤝
.