You need to mark container’s start class using the @YarnComponent
and mark method with the @OnContainerStart
annotations:
@YarnComponent
class YourContainer {
@OnContainerStart
public void onContainerStart() {
// your code
}
}
Despite the fact that the description of the annotation says that it cannot return anything, the return value works. We make the return value of type int.
0 – means successful shutdown of the container, non-zero values – error:
@YarnComponent
class YourContainer {
@OnContainerStart
public int onContainerStart() {
try {
// your code
} catch (Exception e) {
log.error(e.getMessage());
return 1;
}
return 0;
}
}
The return value should be smaller then 256
. You may notice, that:
- If you terminate the container with exit code
0
or some other positive number, then the same code will be sent to AppMaster; - If you terminate the container with a negative code, then another number will be sent to AppMaster.
Why is this happening – let’s talk in this article: https://mchesnavsky.tech/why-the-appmaster-receives-a-different-error-code-from-the-container-spring-boot-yarn.
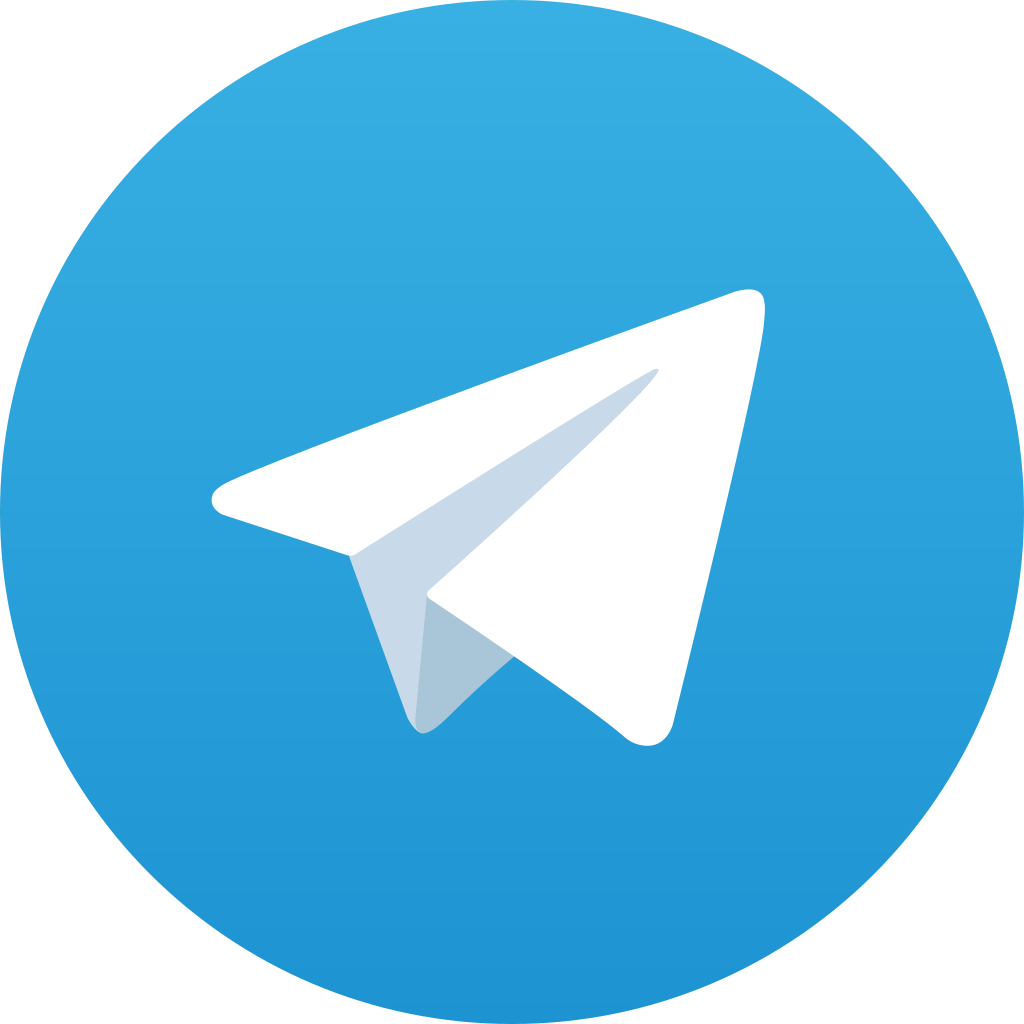
If you still have any questions, feel free to ask me in the comments under this article or write me at promark33@gmail.com.
If I saved your day, you can support me 🤝