gRPC doesn’t support JKS out of the box, but there is a workaround. Below is an example of how to use gRPC and JKS.
Server side
private static final String keyStorePath = "keystore.jks";
private static final String keyStorePass = "secret123";
public void startServer() {
try {
ServerBuilder<?> builder = NettyServerBuilder
.forPort(2185)
.sslContext(buildGRpcSslContext())
.addService(service);
server = builder.build();
server.start();
} catch (Exception e) {
log.error("Can't start gRPC server", e);
}
}
private SslContext buildGRpcSslContext() throws Exception {
log.info("Building gRPC SSL context");
KeyStore keyStore = KeyStore.getInstance("JKS");
keyStore.load(new FileInputStream(keyStorePath), keyStorePass.toCharArray());
KeyManagerFactory keyManagerFactory = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
keyManagerFactory.init(keyStore, keyStorePass.toCharArray());
return GrpcSslContexts.configure(SslContextBuilder.forServer(keyManagerFactory), SslProvider.OPENSSL).build();
}
Client side
If you don’t need to use custom truststore, you need to do nothing. If you need to use custom truststore, use example below:
NettyChannelBuilder.forPort(2185)
.sslContext(GrpcSslContexts.configure(SslContextBuilder.forServer(trustManagerFactory)).build())
.build();
You can use trustManagerFactory
object creation example from the server side code, just use TrustManagerFactory
instead of KeyManagerFactory
.
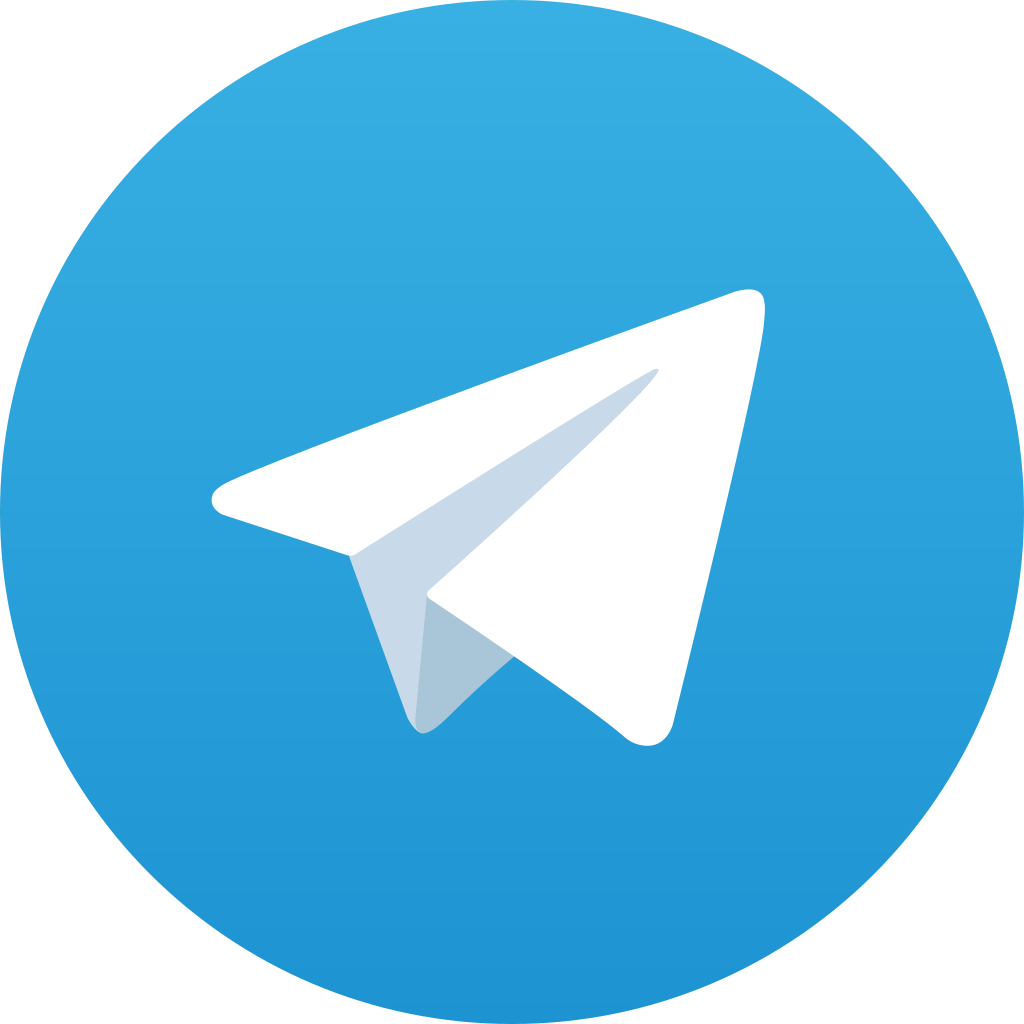
If you still have any questions, feel free to ask me in the comments under this article or write me at promark33@gmail.com.
If I saved your day, you can support me 🤝